BUBBLE SORT
WHAT IS BUBBLE SORT?
Bubble Sort is the simplest sorting algorithm that works by repeatedly swapping the adjacent elements if they are in wrong order.
EXAMPLE:
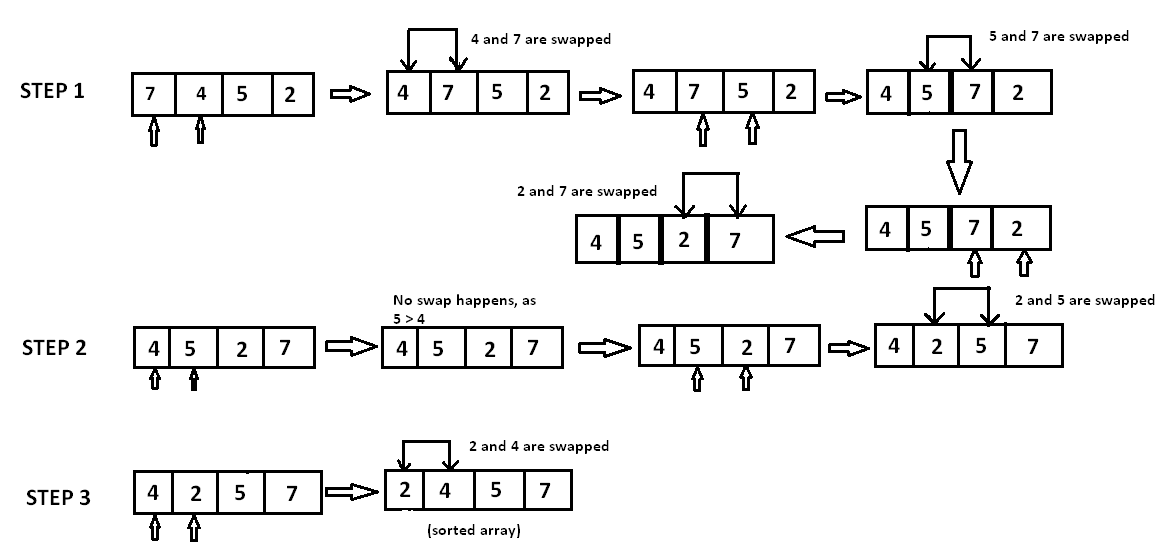
ALGORITHM:
begin BubbleSort(list) for all elements of list if list[i] > list[i+1] swap(list[i], list[i+1]) end if end for return list end BubbleSort
CODE:
#include <stdio.h>
void bubble_sort(int a[], int n) {
int i = 0, j = 0, tmp;
for (i = 0; i < n; i++) { // loop n times - 1 per element
for (j = 0; j < n - i - 1; j++) { // last i elements are sorted already
if (a[j] > a[j + 1]) { // swop if order is broken
tmp = a[j];
a[j] = a[j + 1];
a[j + 1] = tmp;
}
}
}
}
int main() {
int a[100], n, i, d, swap;
printf("Enter number of elements in the array:\n");
scanf("%d", &n);
printf("Enter %d integers\n", n);
for (i = 0; i < n; i++)
scanf("%d", &a[i]);
bubble_sort(a, n);
printf("Printing the sorted array:\n");
for (i = 0; i < n; i++)
printf("%d\n", a[i]);
return 0;
}
WATCH FULL VIDEO ON YOUTUBE
🔻🔻🔻🔻🔻🔻🔻
THANKYOU
RAJ ARYAN